백준 JAVA 1197번 최소 스패닝 트리
문제 링크: https://www.acmicpc.net/problem/1197
1197번: 최소 스패닝 트리
첫째 줄에 정점의 개수 V(1 ≤ V ≤ 10,000)와 간선의 개수 E(1 ≤ E ≤ 100,000)가 주어진다. 다음 E개의 줄에는 각 간선에 대한 정보를 나타내는 세 정수 A, B, C가 주어진다. 이는 A번 정점과 B번 정점이
www.acmicpc.net
최소 스패닝 트리(MST)의 가장 기본적인 문제라고 할 수 있다.
find를 통하여 부모를 찾고, union을 통하여 부모가 다를 경우 합치는 방식이다.
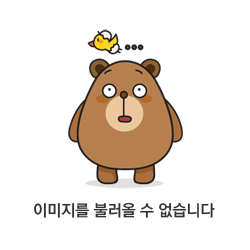
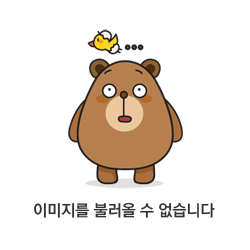
import java.util.*; class Node implements Comparable<Node>{ int start, end, weight; public Node(int start, int end, int weight){ this.start=start; this.end=end; this.weight=weight; } @Override public int compareTo(Node o) { return this.weight-o.weight; } } public class Main { static int [] parent; static PriorityQueue<Node> pq; static int n, k, start, end; public static void main(String[] args){ Scanner sc = new Scanner(System.in); n=sc.nextInt(); k=sc.nextInt(); parent= new int[n+1]; pq= new PriorityQueue<>(); for(int i=1; i<=n; i++){ parent[i]=i; } for(int i=0; i<k; i++){ int a= sc.nextInt(); int b= sc.nextInt(); int c=sc.nextInt(); pq.add(new Node(a,b,c)); } System.out.println(solve()); } private static int solve() { int sum=0; while(!pq.isEmpty()){ Node node = pq.poll(); int parentS = find(node.start); int parentE = find(node.end); if(parentS!=parentE){ Union(parentS,parentE); sum+=node.weight; } } return sum; } private static int find(int x){ if(parent[x]==x){ return x; } else{ return parent[x]=find(parent[x]); } } private static void Union(int a, int b){ a=find(a); b=find(b); if(a!=b){ parent[b]=a; } } }
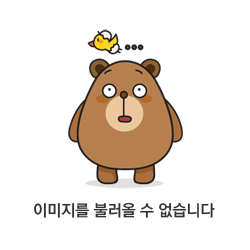
'Alogorithm > Tree' 카테고리의 다른 글
백준 1717[자바] java 집합의 표현 (0) | 2022.02.11 |
---|---|
백준 14675 [자바] java 단절점과 단절선 (0) | 2022.01.18 |
백준 1647 [자바] java 도시 분할 계획 (0) | 2022.01.10 |
백준 JAVA 1992번 네트워크 연결 (0) | 2021.12.20 |
백준 JAVA 1991번 트리 순회 (0) | 2021.12.01 |
댓글
이 글 공유하기
다른 글
-
백준 14675 [자바] java 단절점과 단절선
백준 14675 [자바] java 단절점과 단절선
2022.01.18 -
백준 1647 [자바] java 도시 분할 계획
백준 1647 [자바] java 도시 분할 계획
2022.01.10 -
백준 JAVA 1992번 네트워크 연결
백준 JAVA 1992번 네트워크 연결
2021.12.20 -
백준 JAVA 1991번 트리 순회
백준 JAVA 1991번 트리 순회
2021.12.01
댓글을 사용할 수 없습니다.